Article Series
- Tutorial 1: Angular JS
- Tutorial 2: Controllers
- Tutorial 3: Controllers
- Tutorial 4: Controllers: Advanced
- Tutorial 5: Models
- Tutorial 6: Services
Prerequisite: AngularJS: Controllers: Tutorial 2
In this tutorial, we will look more into how we can segregate Controllers from the Views and also learn on a new directive which will be used quite often in real time. We will first go on and create a simple Book Store application.
We will in the beginning, create an ‘app.js’ file in which we will write our Controller logic.
var Namespace = angular.module('Namespace', []);
Namespace.controller('ControllerName', ['$scope', function($scope) {
$scope.name = "Aditya S",
$scope.bookarray=[
{
bookName : "Book of Eli",
bookCost : 15,
bookImage : 'images/BookStack.png'
},
{
bookName : "Introduction to Angular",
bookCost : 21,
bookImage : 'images/Books.png'
}
]
}]);
In the above snippet, we have created a simple function with a name
property and an array with static inputs.
Our HTML code is very easy and would look like below:
<!DOCTYPE html>
<html lang="en" ng-app="Namespace">
<head>
<meta charset="utf-8">
<title>Hello World</title>
<link rel="stylesheet" type="text/css" href="css/templates.css" />
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.3/angular.min.js">
</script>
<script src="javascript/app.js"></script>
</head>
<body ng-controller="ControllerName as control">
Hello {{name}}
<h1>Welcome to my book store</h1>
<div ng-repeat="arr in bookarray">
<table>
<tr>
<td>
<div>
<img ng-src="{{arr.bookImage}}" />
</div></td>
<td>
<div>
<div>
<b>Book Name :</b>{{arr.bookName}}</div>
<div>
<b>Book Cost :</b>{{arr.bookCost | currency}}</div>
</div></td>
</tr>
</table>
</div>
</body>
</html>
Again, in the above snippet, I am creating a table to display my array. But an interesting fact here is that we are using an Angular inbuilt directive called “ng-repeat
” which would process the array and repeat it for us.
The application would look like:
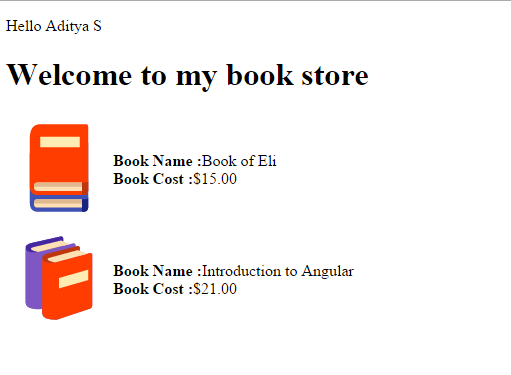
In the next tutorial, we will learn more on creating tabs and their interaction with few other directives.