Introduction
PHPRS (https://github.com/caoym/phprs-restful) is a lightweight, easy-to-use and jax-rs-like open source library for building RESTful Web Services.
Using the Code
Here is an "Order Manager" sample.
class Orders
{
public function getAllOrders() {
return Sql::select('*')->from('orders')->get($this->db);
}
public function getOrderById($id) {
return Sql::select('*')->from('orders')
->where('id=?',$id)->get($this->db);
}
public function createOrder($goods_info){
$order_id = Sql::insertInto('orders')
->values($goods_info)->exec($this->db)->lastInsertId();
return ['order_id'=>$order_id];
}
public $db;
}
PHPRS use annotations like @path
,@route
to define routers, two-way parameter binding, dependency injection, etc.
Features
Flexible Routes
PHPRS use @route
to define routes.
@route({"GET","/patha"}) | GET | /patha, /patha/...
------------------------------------------+-----------+---------------------
@route({"*","/patha"}) | GET | /patha
| POST |
| PUT |
| DELETE |
| HEAD |
| ... |
------------------------------------------+-----------+---------------------
@route({"GET","\patha\*\pathb"}) | GET | /patha/xxx/pathb
------------------------------------------+-----------+---------------------
@route({"GET", "func1?param1=1¶m2=2"})| GET | /func1?param1=1¶m2=2&...
| | /myapi/func1?param2=2¶m1=1&...
Two-way Parameter Binding
Annotations: @param
, @return
, @throws
is used to bind variables between function parameters and http request or response.
------------------------------------------+-----------------------------
@param({"arg0","$._GET.arg0"}) | $arg0 = $_GET['arg0']
------------------------------------------+-----------------------------
@param({"arg1","$.path[1]"}) | $arg1 = explode('/', REQUEST_URI)[1]
------------------------------------------+-----------------------------
@return({"cookie","token","$arg2"}) | setcookie('token', $arg2)
function testCookie(&$arg2) |
------------------------------------------+-----------------------------
@return({"body"}) | use function return as http response body
------------------------------------------+-----------------------------
@throws({"MyException", | try{}
"res", | catch(MyException) {
"400 Bad Request", | header("HTTP/1.1 400 Bad Request");
body(["error"=>"my exception"]);}
{"error":"my exception"}})
API Cache
Use @cache
to enable cache for this method. If all params of the method are identical, the following calls will use cache.
----------------------------------+-----------------------------
@cache({"ttl",3600}) | set cache as fixed time expire, as ttl 1 hour.
------------------------------------------+-----------------------------
@cache({"checker", "$checker"}) | Use dynamic strategy to check caches.
| $checker is set in method, and will be invoked to check
cache expired with $checker($data, $create_time),
for examples use $check = new FileExpiredChecker('file.tmp');
to make cache invalidated if file.tmp modified.
Dependency Injection
Use @property
to inject dependency PHPRS create API class and inject dependency from conf.json, which looks like:
{
"Orders":{
"properties": {
"db":"@db"
}
},
"db":{
"singleton":true,
"class":"PDO",
"pass_by_construct":true,
"properties":{
"dsn":"mysql:host=127.0.0.1;dbname=testdb;",
"username":"test",
"passwd":"test"
}
}
}
Document Automatic Generation
The document looks like: 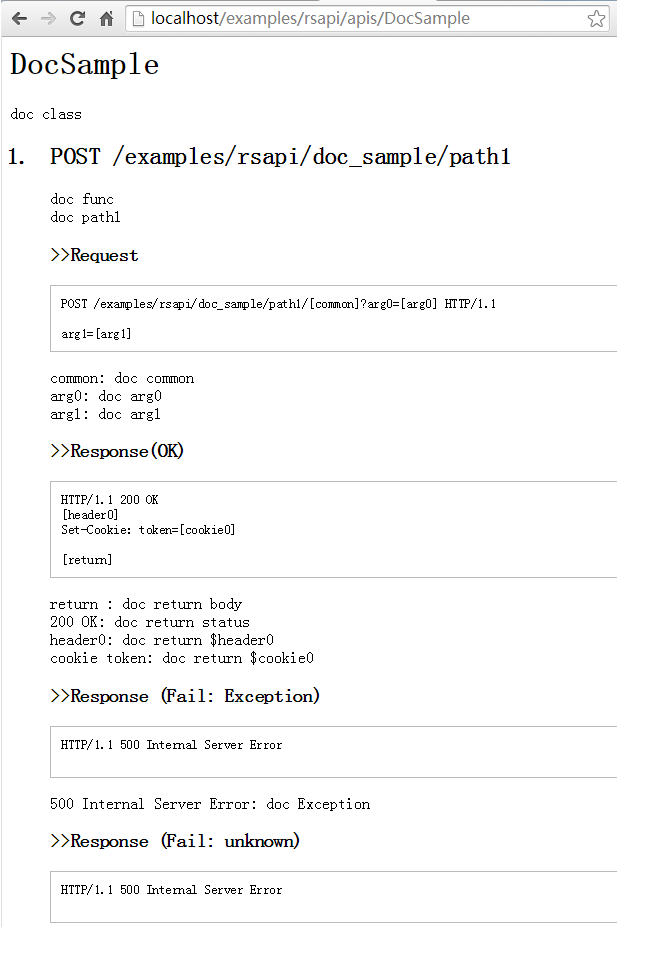
Hook
The implement of a hook is the same as API.
ezsql
An easy-to-use and IDE friendly SQL builder. Object-oriented SQL. @see https://github.com/caoym/ezsql